Rules
Create a Configuration instance
Call the create() method of HBaseConfiguration to instantiate this class. Otherwise, the HBase configurations cannot be successfully loaded.
Correct:
//This part is declared in the class member variable declaration. private Configuration hbaseConfig = null; //Instantiate this class using its constructor function or initialization method. hbaseConfig = HBaseConfiguration.create();
Incorrect:
hbaseConfig = new Configuration();
Share the Configuration instance
The HBase client codes obtain rights to interact with an HBase cluster by creating an HConnection with Zookeeper. Each HConnection has a Configuration instance. The created HConnection instances are cached. That is, if the HBase client needs to communicate with an HBase cluster, the client sends a Configuration instance to the cluster. Then, the HBase client checks for an HConnection instance for the Configuration instance in the cache. If a match is found, the HConnection instance is returned. If no match is found, an HConnection instance will be created.
If the Configuration instance is frequently created, a lot of unnecessary HConnection instances will be created, causing the number of connections to Zookeeper to reach the upper limit.
Therefore, it is recommended that the client codes share the same Configuration instance.
Create an Table instance
public abstract class TableOperationImpl { private static Configuration conf = null; private static Connection connection = null; private static Table table = null; private static TableName tableName = TableName.valueOf("sample_table"); public TableOperationImpl() { init(); } public void init() { conf = ConfigurationSample.getConfiguration(); try { connection = ConnectionFactory.createConnection(conf); table = conn.getTable(tableName); } catch (IOException e) { e.printStackTrace(); } } public void close() { if (table != null) { try { table.close(); } catch (IOException e) { System.out.println("Can not close table."); } finally { table = null; } } if (connection != null) { try { connection.close(); } catch (IOException e) { System.out.println("Can not close connection."); } finally { connection = null; } } } public void operate() { init(); process(); close(); } }
An Table instance cannot be used by multiple threads at the same time
Table is not thread safe for reads or write. If an Table instance is used by multiple threads at the same time, exceptions will occur.
Cache a frequently used Table instance
Cache the Table instance that will be frequently used by a thread for a long period of time. A cached instance, however, will not be necessarily used by a thread permanently. In special circumstances, you need to rebuild an Table instance. See the next rule for details.
Correct:
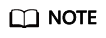
In this example, the Table instance is cached by Map. This method applies when multiple threads and Table instances are required. If an Table instance is used by only one thread and the thread has only one Table instance, Map need not be used.
//In this Map, TableName is the Key value. Cache all Table instances. private Map<String, Table> demoTables = new HashMap<String, Table>(); //All Table instances share this Configuration instance. private Configuration demoConf = null; /** * <Initialize an HTable class> * <Detailed function description> * @param tableName * @return * @throws IOException * @see [class, class#method, class#member] */ private Table initNewTable(String tableName) throws IOException { try (Connection conn = ConnectionFactory.createConnection(demoConf)){ return conn.getTable(tableName); } } /** * <Obtain Table instances> * <Detailed function description> * @see [class, class#method, class#member] */ private Table getTable(String tableName) { if (demoTables.containsKey(tableName)) { return demoTables.get(tableName); } else { Table table = null; try { table = initNewTable(tableName); demoTables.put(tableName, table); } catch (IOException e) { // TODO Auto-generated catch block e.printStackTrace(); } return table; } } /** * <Write data> * <Multi-thread multi-Table instance design optimization is not involved. The synchronization method is used * because the Table is not thread safe. It is recommended that an Table instance be used by only one data write thread at the same *time.> * @param dataList * @param tableName * @see [class, class#method, class#member] */ public void putData(List<Put> dataList, String tableName) {Table table = getTable(tableName); //Synchronization is not required if the Table instance is not shared by multiple threads. //Note that Table is not thread safe. synchronized (table) { try { table.put(dataList); table.notifyAll(); } catch (IOException e) { // When IOE is detected, the cached instance needs to be re-created. try { // Close the Connection. table.close(); // Re-create the instance. table = initNewTable(tableName); } catch (IOException e1) { // TODO } } } }
Incorrect:
public void putDataIncorrect(List<Put> dataList, String tableName) {Table table = null; try { //Create an HTable instance each time when data is written. table = initNewTable(tableName); table.put(dataList); } catch (IOException e1) { // TODO Auto-generated catch block e1.printStackTrace(); } finally { table.close(); } }
Rebuild an Table instance
Rebuilt a cached Table when IOException is detected. See the example of the previous rule.
Do not call the following methods unless necessary:
- Configuration#clear
Do not call this method if a Configuration is used by an object or a thread. The Configuration#clear method clears all attributes loaded. If this method is called for a Configuration used by Table, all the parameters of this Configuration will be deleted from Table. As a result, an exception occurs when Table uses the Configuration the next time.
Therefore, avoid calling this method each time you rebuild an Table instance. Call this method when all the threads need to quit.
- HConnectionManager#deleteAllConnections
This method deletes all connections from the Connection set. As the Table stores the links to the connections, the connections being used cannot be stopped after the HConnectionManager#deleteAllConnections method is called, which eventually causes information leakage.
Handle the data failed to write
Some data write operations may fail due to instant exceptions or process failures. Therefore, the data must be recorded so that it can be written to the HBase when the cluster is restored.
The failed data returned by the HBase client will not be automatically rewritten. The interface caller is only informed of the data failed to be written. To prevent data loss, measures must be taken to temporarily save the data in a file or in memory.
Correct:
private List<Row> errorList = new ArrayList<Row>(); /** * <Insert data in PutList mode. > * <Synchronization is not required if the method is not called by multiple threads.> * @param put a data record * @throws IOException * @see [class, class#method, class#member] */ public synchronized void putData(Put put) { // Temporarily cache data in this List. dataList.add(put); // Perform a Put operation when the dataList size reaches PUT_LIST_SIZE. if (dataList.size() >= PUT_LIST_SIZE) { try { demoTable.put(dataList); } catch (IOException e) { // If RetriesExhaustedWithDetailsException occurs, // certain data failed to be written, which // is caused by process errors in the HBase cluster or migration of a large number of // Regions. if (e instanceof RetriesExhaustedWithDetailsException) { RetriesExhaustedWithDetailsException ree = (RetriesExhaustedWithDetailsException)e; int failures = ree.getNumExceptions(); for (int i = 0; i < failures; i++) { errorList.add(ree.getRow(i)); } } } dataList.clear(); } }
Release resources
Call the Close method to release resources when the ResultScanner and Table instances are not required. To enable the Close method to be called, add the Close method to the finally block.
Correct:
ResultScanner scanner = null; try { scanner = demoTable.getScanner(s); //Do Something here. } finally { scanner.close(); }
Incorrect:
- The code does not call the scanner.close() method to release resources.
- The scanner.close() method is not placed in the finally block.
ResultScanner scanner = null; scanner = demoTable.getScanner(s); //Do Something here. scanner.close();
Add fault-tolerance mechanism for Scan
Exceptions, such as lease expiration, may occur when Scan is performed. Retry operations need to be performed when exceptions occur.
Retry operations can be applied in HBase-related interface methods to improve fault tolerance capabilities.
Stop Admin as soon as it is not required
Stop Admin as soon as possible. Do not cache the same Admin instance for an extended period of time.
Feedback
Was this page helpful?
Provide feedbackThank you very much for your feedback. We will continue working to improve the documentation.