Creating a Table
Function
Use the KuduClient.createTable(String name, Schema schema, CreateTableOptions builder) method to create a table object, in which the schema and partition information of the table must be specified.
Sample Code
The code snippet for creating a table is as follows:
// Set up a table name. String tableName = "example"; // Set up a simple schema. List<ColumnSchema> columns = new ArrayList<>(2); columns.add(new ColumnSchema.ColumnSchemaBuilder("key", Type.INT32) .key(true) .build()); columns.add(new ColumnSchema.ColumnSchemaBuilder("value",Type.STRING).nullable(true) .build()); Schema schema = new Schema(columns); // Set up the partition schema, which distributes rows to different tablets by hash. // Kudu also supports partitioning by key range. Hash and range partitioning can be combined. // For more information, see http://kudu.apache.org/docs/schema_design.html. CreateTableOptions cto = new CreateTableOptions(); List<String> hashKeys = new ArrayList<>(1); hashKeys.add("key"); int numBuckets = 8; cto.addHashPartitions(hashKeys, numBuckets); // Create the table. client.createTable(tableName, schema, cto);
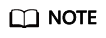
In the sample code, a table is defined, including the key and value columns. key is a primary key field of the int32 type, and value is a non-primary key field of the string type and can be empty. In addition, eight hash partitions are created for the key field, indicating that the data is divided into eight independent tablets.
Feedback
Was this page helpful?
Provide feedbackThank you very much for your feedback. We will continue working to improve the documentation.