C# Function Development
Function Syntax
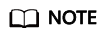
You are advised to use .NET Core 3.1.
FunctionGraph supports C# (.NET Core 2.1) and C# (.NET Core 3.1).
Scope Return parameter Function name (User-defined parameter, Context)
- Scope: It must be defined as public for the function that FunctionGraph invokes to execute your code.
- Return parameter: user-defined output, which is converted into a character string and returned as an HTTP response.
- Function name: user-defined function name. The name must be consistent with that you define when creating a function.
In the navigation pane on the left of the FunctionGraph console, choose Functions > Function List. Click the name of the function to be set. On the function details page that is displayed, choose Configuration > Basic Settings and set the Handler parameter, as shown in Figure 1. The parameter value is CsharpDemo::CsharpDemo.Program::MyFunc (format: [assembly]::[namespace].[class name]::[execution function name]).
- Event: event parameter defined for the function.
- context: runtime information provided for executing the function. For details, see the description of SDK APIs.
The HC.Serverless.Function.Common library needs to be referenced when you deploy a project in FunctionGraph. For details about the IFunctionContext object, see the context description.
When creating a C# function, you need to define a method as the handler of the function. The method can access the function by using specified IFunctionContext parameters. Example:
1 2 3 4
public Stream handlerName(Stream input,IFunctionContext context) { // TODO }
Function Handler
ASSEMBLY::NAMESPACE.CLASSNAME::METHODNAME
- ASSEMBLY: name of the .NET assembly file for your application, for example, HelloCsharp.
- NAMESPACE and CLASSNAME: names of the namespace and class to which the handler function belongs.
- METHODNAME: name of the handler function. Example:
Set the handler to HelloCsharp::Example.Hello::Handler when you create a function.
SDK APIs
- Context APIs
Table 1 describes the provided context attributes.
Table 1 Context objects Attribute
Description
String RequestId
Request ID.
String ProjectId
Project Id
String PackageName
Name of the group to which the function belongs.
String FunctionName
Function name.
String FunctionVersion
Function version.
Int MemoryLimitInMb
Allocated memory.
Int CpuNumber
CPU usage of a function.
String Accesskey
Obtains the AK (valid for 24 hours) with an agency. If you use this method, you need to configure an agency for the function.
NOTE:FunctionGraph has stopped maintaining the String AccessKey API in the Runtime SDK. You cannot use this API to obtain a temporary AK.
String Secretkey
Obtains the SK (valid for 24 hours) with an agency. If you use this method, you need to configure an agency for the function.
NOTE:FunctionGraph has stopped maintaining the String SecretKey API in the Runtime SDK. You cannot use this API to obtain a temporary SK.
String SecurityAccessKey
Obtains the SecurityAccessKey (valid for 24 hours) with an agency. If you use this method, you need to configure an agency for the function.
String SecuritySecretKey
Obtains the SecuritySecretKey (valid for 24 hours) with an agency. If you use this method, you need to configure an agency for the function.
String SecurityToken
Obtains the SecurityToken (valid for 24 hours) with an agency. If you use this method, you need to configure an agency for the function.
String Token
Obtains the token (valid for 24 hours) with an agency. If you use this method, you need to configure an agency for the function.
Int RemainingTimeInMilliSeconds
Remaining running time of a function.
String GetUserData(string key,string defvalue=" ")
Uses keys to obtain the values passed by environment variables.
- Logging APIs
The following table describes the logging APIs provided in the C# SDK.
Table 2 Logging APIs Method
Description
Log(string message)
Creates a logger object by using context.
var logger = context.Logger;
logger.Log("hello CSharp runtime test(v1.0.2)");
Logf(string format, args ...interface{})
Creates a logger object by using context.
var logger = context.Logger;
var version = "v1.0.2"
logger.Logf("hello CSharp runtime test({0})", version);
Execution Result
The execution result consists of the function output, summary, and log output.
Parameter |
Successful Execution |
Failed Execution |
---|---|---|
Function Output |
The defined function output information is returned. |
A JSON file that contains errorMessage and errorType is returned. The format is as follows: { "errorMessage": "", "errorType": "" } errorMessage: Error message returned by the runtime. errorType: Error type. |
Summary |
Request ID, Memory Configured, Execution Duration, Memory Used, and Billed Duration are displayed. |
Request ID, Memory Configured, Execution Duration, Memory Used, and Billed Duration are displayed. |
Log Output |
Function logs are printed. A maximum of 4 KB logs can be displayed. |
Error information is printed. A maximum of 4 KB logs can be displayed. |
Feedback
Was this page helpful?
Provide feedbackThank you very much for your feedback. We will continue working to improve the documentation.