Setting or Obtaining a Versioning Object ACL
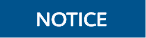
If you have any questions during development, post them on the Issues page of GitHub. For details about parameters and usage of each API, see API Reference.
Directly Setting a Versioning Object ACL
You can call ObsClient->setObjectAcl to set the ACL for a versioning object by specifying the version ID (VersionId). Sample code is as follows:
// Import the dependency library. require 'vendor/autoload.php'; // Import the SDK code library during source code installation. // require 'obs-autoloader.php'; // Declare the namespace. use Obs\ObsClient; // Create an instance of ObsClient. $obsClient = new ObsClient ( [ //Obtain an AK/SK pair using environment variables or import the AK/SK pair in other ways. Using hard coding may result in leakage. //Obtain an AK/SK pair on the management console. For details, see https://support.huaweicloud.com/eu/usermanual-ca/ca_01_0003.html. 'key' => getenv('ACCESS_KEY_ID'), 'secret' => getenv('SECRET_ACCESS_KEY'), 'endpoint' => 'https://your-endpoint' ] ); $resp = $obsClient -> setObjectAcl([ 'Bucket' => 'bucketname', 'Key' => 'objectname', 'VersionId' => 'versionid', // Set the versioning object ACL to public-read by specifying the pre-defined access control policy. 'ACL' => ObsClient::AclPublicRead ]); printf("RequestId:%s\n", $resp['RequestId']); $resp = $obsClient -> setObjectAcl([ 'Bucket' => 'bucketname', 'Key' => 'objectname', 'VersionId' => 'versionid', // Set the object owner. 'Owner' => ['ID' => 'ownerid'], 'Grants' => [ // Grant the READ permission to all users. ['Grantee' => ['Type' => 'Group', 'URI' => ObsClient::GroupAllUsers], 'Permission' => ObsClient::PermissionRead], ] ]); printf("RequestId:%s\n", $resp['RequestId']);
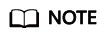
- Use the Owner parameter to specify the object owner and the Grants parameter to specify information about the authorized users.
- The owner or grantee ID needed in the ACL indicates the account ID, which can be viewed on the My Credentials page of OBS Console.
- OBS buckets support the following grantee group:
- All users: ObsClient::GroupAllUsers
Obtaining a Versioning Object ACL
You can call ObsClient->getObjectAcl to obtain the ACL of a versioning object by specifying the version ID (VersionId). Sample code is as follows:
// Import the dependency library. require 'vendor/autoload.php'; // Import the SDK code library during source code installation. // require 'obs-autoloader.php'; // Declare the namespace. use Obs\ObsClient; // Create an instance of ObsClient. $obsClient = new ObsClient ( [ //Obtain an AK/SK pair using environment variables or import the AK/SK pair in other ways. Using hard coding may result in leakage. //Obtain an AK/SK pair on the management console. For details, see https://support.huaweicloud.com/eu/usermanual-ca/ca_01_0003.html. 'key' => getenv('ACCESS_KEY_ID'), 'secret' => getenv('SECRET_ACCESS_KEY'), 'endpoint' => 'https://your-endpoint' ] ); $resp = $obsClient->getObjectAcl ( [ 'Bucket' => 'bucketname', 'Key' => 'objectname', 'VersionId' => 'versionid' ] ); printf ( "RequestId:%s\n", $resp ['RequestId'] ); printf ( "Owner[ID]:%s\n", $resp ['Owner'] ['ID'] ); foreach ( $resp ['Grants'] as $index => $grant ) { printf ( "Grants[%d]\n", $index + 1 ); printf ( "Grantee[ID]:%s\n", $grant ['Grantee'] ['ID'] ); printf ( "Grantee[URI]:%s\n", $grant ['Grantee'] ['URI'] ); printf ( "Permission:%s\n", $grant ['Permission'] ); }
Feedback
Was this page helpful?
Provide feedbackThank you very much for your feedback. We will continue working to improve the documentation.