Merging Game Servers with DCS
Scenario
Merging game servers is a strategy for some large online games. After running a game for a while, game providers set up a new server to attract new players. As users shift to the new server, game developers usually merge the new server and the old one, so new and old players can play together for a better game experience. During this process, game developers must consider how to synchronize data among different servers.
Solution
DCS for Redis can be used in the following game server merge scenarios:
- Cross-server data synchronization
After servers merger, data on multiple servers needs to be synchronized to ensure consistency. With the pub/sub message queuing mechanism of Redis, data changes can be published to Redis channels. Other game servers can subscribe to the channels to receive messages of changes.
- Cross-server resource sharing
After servers merge, resources on multiple servers, such as player props and gold coins, can be shared. The distributed lock mechanism of Redis can ensure mutual exclusion among multiple servers in resource access.
- Cross-server ranking
After servers merge, rankings on multiple servers can be combined to show the ranking over all servers. Sorted sets in Redis can store ranking data and perform calculation and query.
For details about cross-server resource sharing, see Serializing Access to Frequently Accessed Resources. For details about cross-server ranking, see Ranking with Redis.
The following describes how to implement cross-server data synchronization through pub/sub message queuing in Redis.
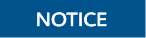
When using Redis for game server merge, you need to consider data consistency, performance, and security. Issues such as data errors, performance bottlenecks, and security vulnerabilities should be avoided.
Procedure
- Use the Redis() method from the redis-py library to create a Redis client connection on each game server.
- Use the pubsub() method to create a Redis subscriber and publisher on each game server. They will be used for subscribing to messages from other game servers and publishing data changes on the local server. When a server needs to update data, it publishes updates to the Redis message queue. Other servers will receive the updates and update their local data.
- Define a publish_update() method to publish updates, and use the subscriber.listen() method in the listen_updates() method to listen to updates.
- Once an update is captured, the handle_update() method is invoked to process the update and update local data. In game servers, the publish_update() method can be invoked to publish updates, and the listen_updates() method can be invoked to listen to updates.
Sample Code
The sample code for using the pub/sub mechanism to implement cross-server game data synchronization is as follows:
import redis # Create a Redis client connection. redis_client = redis.Redis(host='localhost', port=6379, db=0) # Create a subscriber. subscriber = redis_client.pubsub() subscriber.subscribe('game_updates') # Create a publisher. publisher = redis_client # Publish updates. def publish_update(update): publisher.publish('game_updates', update) # Process updates. def handle_update(update): # Update local data. print('Received update:', update) # Listen to updates. def listen_updates(): for message in subscriber.listen(): if message['type'] == 'message': update = message['data'] handle_update(update) # Invoke publish_update(). publish_update('player_data_updated') # Invoke listen_updates(). listen_updates()
Result:
D:\workspace\pythonProject\venv\Scripts\python.exe D:\workspace\pythonProject\test2.py Received update: b'player_data_updated'
Feedback
Was this page helpful?
Provide feedbackThank you very much for your feedback. We will continue working to improve the documentation.