GET Object
API Description
You can use this API to download an object from a specified bucket.
Method Definition
ObsClient.getObject
Request Parameter
Field |
Type |
Optional or Mandatory |
Description |
---|---|---|---|
Bucket |
String |
Mandatory |
Bucket name |
Key |
String |
Mandatory |
Object name |
RequestDate |
String or Date |
Optional |
Request time
NOTE:
When the parameter type is String, the value must comply with the ISO8601 or RFC822 standards. |
VersionId |
String |
Optional |
Object version ID |
IfMatch |
String |
Optional |
Returns the source object if its ETag is the same as the one specified by this parameter; otherwise, an error code is returned. |
IfModifiedSince |
String |
Optional |
Returns the object if it is modified after the time specified by this parameter; otherwise, an error code is returned. The value must conform to the HTTP time format specified in http://www.ietf.org/rfc/rfc2616.txt. |
IfNoneMatch |
String |
Optional |
Returns the source object if its ETag is different from the one specified by this parameter; otherwise, an error code is returned. |
IfUnmodifiedSince |
String |
Optional |
Returns the object if it remains unchanged since the time specified by this parameter; otherwise, an exception is thrown. The value must conform to the HTTP time format specified in http://www.ietf.org/rfc/rfc2616.txt. |
Range |
String |
Optional |
Download range. The value range is [0, object length-1] and is in the format of bytes = x-y. The maximum length of Range is the length of the object minus 1. If it exceeds this value, the length of the object minus 1 is used. |
Origin |
String |
Optional |
Origin of the cross-origin request specified by the preflight request. Generally, it is a domain name. |
RequestHeader |
String |
Optional |
HTTP header in a cross-domain request |
ResponseCacheControl |
String |
Optional |
Rewrites the Cache-Control header in the response. |
ResponseContentDisposition |
String |
Optional |
Rewrites the Content-Disposition header in the response. |
ResponseContentEncoding |
String |
Optional |
Rewrites the Content-Encoding header in the response. |
ResponseContentLanguage |
String |
Optional |
Rewrites the Content-Language header in the response. |
ResponseContentType |
String |
Optional |
Rewrites the Content-Type header in the response. |
ResponseExpires |
String |
Optional |
Rewrites the Expires header in the response. |
SaveByType |
String |
Optional |
Storage class of the object. Possible values are:
|
ProgressCallback |
Function |
Optional |
Callback function for obtaining the download progress
NOTE:
This callback function contains the following parameters in sequence: Number of downloaded bytes, total bytes, and used time (unit: second). |
SseC |
String |
Optional |
Algorithm used in SSE-C decryption. The value can be:
|
SseCKey |
String |
Optional |
Key used in SSE-C decryption, which is calculated by using AES-256. |
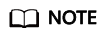
- If the download request includes IfUnmodifiedSince or IfMatch and IfUnmodifiedSince or IfMatch is not met, an exception will be thrown with HTTP status code 412 Precondition Failed.
- If the download request includes IfModifiedSince or IfNoneMatch and IfModifiedSince or IfNoneMatch is not met, an exception will be thrown with HTTP status code 304 Not Modified.
Returned Result (InterfaceResult)
Field |
Type |
Description |
---|---|---|
RequestId |
String |
Request ID returned by the OBS server |
DeleteMarker |
String |
Whether the deleted object is a delete marker |
LastModified |
String |
Time when the last modification was made to the object |
ContentLength |
String |
Object size in bytes |
CacheControl |
String |
Cache-Control header in the response |
ContentDisposition |
String |
Content-Disposition header in the response |
ContentEncoding |
String |
Content-Encoding header in the response |
ContentLanguage |
String |
Content-Language header in the response |
ContentType |
String |
MIME type of the object |
Expires |
String |
Expires header in the response |
ETag |
String |
Object ETag |
VersionId |
String |
Object version ID |
WebsiteRedirectLocation |
String |
Location where the object is redirected to, when the bucket is configured with website hosting |
StorageClass |
String |
Storage class of the object. When the storage class is OBS Standard, the value is null. |
Restore |
String |
Restore status of the object in the Archive storage class |
Expiration |
String |
Expiration details |
Content |
String or ArrayBuffer or Blob or Object |
Object content. If the SavaByType parameter is not set or is set to text, the value of this parameter is a String object. If the SavaByType parameter is set to arraybuffer, the value of this parameter is an ArrayBuffer object. If the SavaByType parameter is set to blob, the value of this parameter is a Blob object. If the SavaByType parameter is set to file, the value is an Object object, representing the URL of the object (URL validity period: 3600 seconds). |
Metadata |
Object |
Customized metadata of the object You need to add the additional headers allowed to be carried in the response in the CORS configurations. For example, you can add the x-amz-meta-property1 header to obtain customized metadata property1. |
Sample Code
obsClient.getObject({ Bucket : 'bucketname', Key : 'objectkey', Range: 'bytes=0-10', SaveByType: 'file' },function (err, result) { if(err){ console.error('Error-->' + err); }else{ if(result.CommonMsg.Status < 300){ console.log('RequestId-->' + result.InterfaceResult.RequestId); console.log('ETag-->' + result.InterfaceResult.ETag); console.log('VersionId-->' + result.InterfaceResult.VersionId); console.log('ContentLength-->' + result.InterfaceResult.ContentLength); console.log('DeleteMarker-->' + result.InterfaceResult.DeleteMarker); console.log('LastModified-->' + result.InterfaceResult.LastModified); console.log('StorageClass-->' + result.InterfaceResult.StorageClass); console.log('Content-->' + result.InterfaceResult.Content); console.log('Metadata-->' + JSON.stringify(result.InterfaceResult.Metadata)); }else{ console.log('Code-->' + result.CommonMsg.Code); console.log('Message-->' + result.CommonMsg.Message); } } });
Feedback
Was this page helpful?
Provide feedbackThank you very much for your feedback. We will continue working to improve the documentation.